Sequentum Cloud API Usage
The Sequentum Cloud API is designed for simplicity and ease of use, enabling users to integrate and interact with their agents seamlessly without going through the Sequentum dashboard.
Integrating with Sequentum Cloud API first requires an API key. First, click on the “Organization” tab in the bottom left of the dashboard.
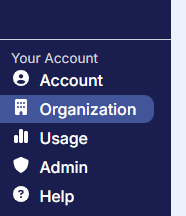
Organization Button
On the “Users” tab, locate the column labeled “API Keys” and you should see a “0” in that column by default next to your email. Click on the “0” to be redirected to the API key creation page.

Users Screen
On the API key creation page, click on “New API Key” in the top right corner to create a new API Key.

API Key Page
Add in a description (optional) or extend the expiration date (optional) and press “Add Key”.
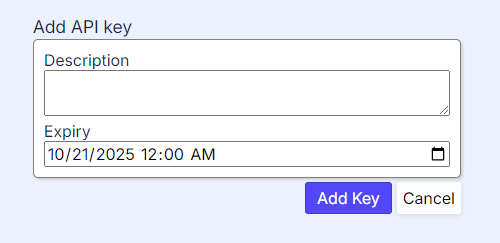
Adding an API Key
An API key has now been created under the “Key” tab and can be used in your API requests.

API Key successfully added
Example of a working API Request:https://dashboard.sequentum.com/api/external/run/load-page?key={insert_api_key_here}&path={path_of_your_agent}