Export Script
Export Script: The “ Export Script” command is available under the “Export” commands section. The command is used for executing custom scripts as needed. Export scripts can be used to customize the export process. An export script could be used to export extracted data to custom data structures in a database or it could export data to a custom data source. There can be various use cases of custom scripting in an agent. The command also provides the option of selecting a programming language to write a custom script with your preferred language.
The Export Script command is located under the Export option in the command palette (refer the below snapshot)
In order to make the optimum use of the Export Script command, we can configure the options as shown in the below screenshot, as per the user requirement.
Command Configuration
The configuration screen for the Export Script command has two tabs viz. Options and Text. Use the ‘Options’ tab to set the command name and other command properties. Use the ‘Text’ tab to navigate to the text of the agent where the command part is present.
Command Properties
On the Options tab of the command, we have three options:
General Settings
Script
Files

General Settings → General Command Settings
This section allows users to customize the command with the following properties:
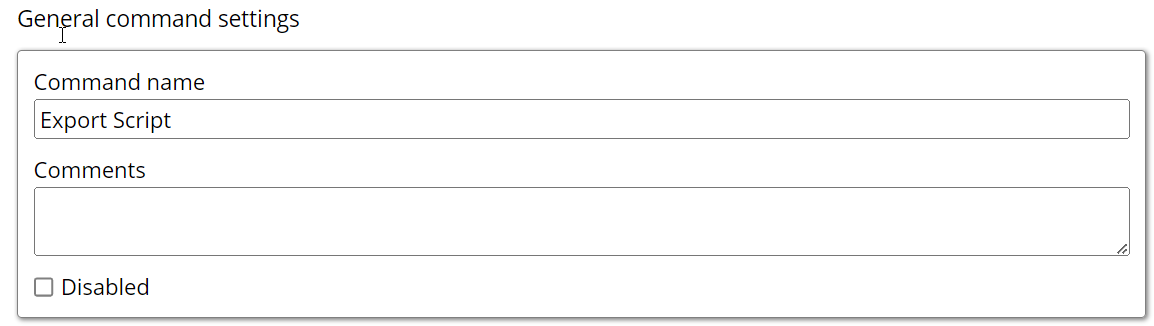
Command name: This property specifies the name of the command.
Comments: Provides a field for entering metadata or a brief description regarding the command. These annotations are used internally to provide context for developers or team members. Comments are not part of the exported data and are only used for documentation purposes within the configuration.
Disabled: This checkbox allows the user to disable the command. When checked, the command is ignored during execution. By default, it is unchecked.
Script
This option provides the feature to write custom scripts with the help of the available languages that are supported by the Sequentum Cloud from the drop-down. It also provides the feature to add or set the timeout for your custom script and a test button to check whether the script is working as expected or not.
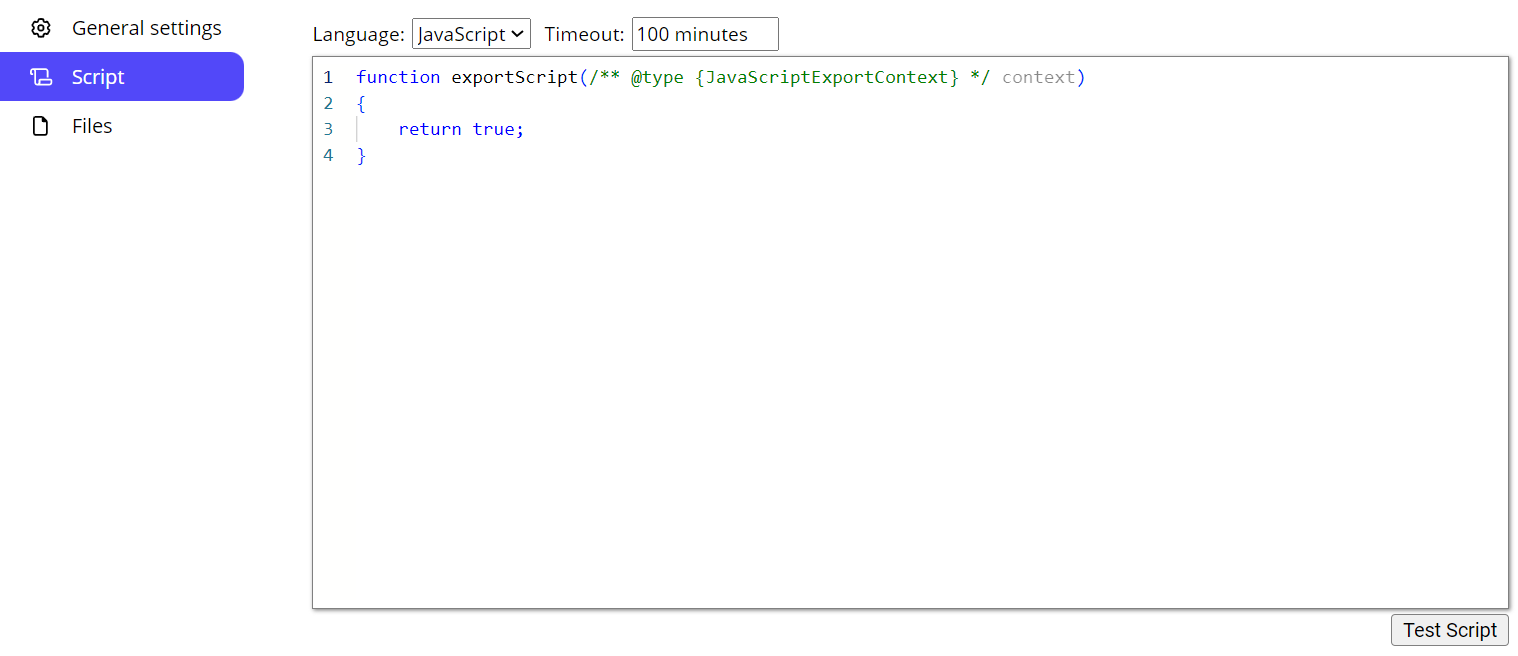
Language: It provides the list of programming languages supported by Sequentum Cloud which are as below:
Javascript
C#
Python
You can select the language of your choice from the above list and start scripting. The Default Language is “Javascript”.

C# example script->This script automates the process of monitoring sentiment in news articles, formatting the results into an email and dispatching alerts when negative sentiment is detected.
Code
#r System.Data.Common.dll
#r System.Data.dll
#r System.Net.dll
#r System.Net.Mail.dll
#r System.ComponentModel.TypeConverter.dll
#r System.Net.Primitives.dll
#r System.Runtime.InteropServices.dll
using System;
using System.IO;
using System.Data;
using System.Text;
using System.Net;
using System.Net.Mail;
using System.Collections.Generic;
public static bool ExportScript(ExportContext context)
{
var ChatGPT= context.GlobalData["ChatGPT"] as string;
if(ChatGPT == "1")
{
context.Log("Executing Email script");
var data_files = new List<string>();
data_files = context.ExportFiles.GetDataFiles();
var filename= data_files[0];
var EXPORT_DIR= Path.GetDirectoryName(filename);
var processedFileName = Path.Combine(EXPORT_DIR, "processed_articles.csv");
//context.Log("ProcessedFile "+processedFileName);
if (File.Exists(processedFileName) && (new FileInfo(processedFileName).Length!= 0))
{
context.Log("DataTable is not empty");
var emailBody = generateEmailBody(context, processedFileName);
//context.Log("emailBody"+emailBody.ToString());
var dtemail = DateTime.UtcNow.ToString("MM-dd-yyyy");
string subject = "V4 NewsMonitorBing- Negative Sentiment Alert:" + dtemail;
sent_email(context, subject, emailBody);
}
else
{
context.Log("DataTable is empty");
return true;
}
}
return true;
}
public static void sent_email(ExportContext context, string emailSubject, string emailBody)
{
if (!string.IsNullOrEmpty(emailBody))
{
var toAddress = context.GlobalData["DistributionList"] as string;
context.Log(toAddress);
var dtemail = DateTime.UtcNow.ToString("MM-dd-yyyy");
context.Log("Successful Credentials");
string img1=context.SharedFiles["NewsMonitor.zip/1c195a45eb5b931303b1766dc9854dbf.png"];
string img2=context.SharedFiles["NewsMonitor.zip/81d966296b73f2aeecc892b90a47710b.jpg"];
string img3=context.SharedFiles["NewsMonitor.zip/465665d51ef28198d2939afe39b25604.png"];
string img4=context.SharedFiles["NewsMonitor.zip/8368706fdbd631efccf7eb83699d3214.png"];
string[] imagesList = {img1, img2, img3, img4};
try
{
context.ScriptUtilities.SendEmail(toAddress, emailSubject, emailBody, true, null, null, null, imagesList, true, false);
//smtp.Send(message);
context.Log("Email sent successfully!");
}
catch (Exception ex)
{
context.Log("Error sending email: " + ex.Message);
}
}
}
public static string generateEmailBody(ExportContext context, string csvPath)
{
//context.Log("Under generateEmailBody function");
Dictionary<string, List<Dictionary<string, string>>> negativeNewsArticles = new Dictionary<string, List<Dictionary<string, string>>>();
List<string> emailMessageList = new List<string>();
var articleOpeningTag = @"<div class=""w pr-16 pl-16"" style=""background:#fffffe;background-color:#fffffe;margin:0px auto;max-width:622px;"">
<table align=""center"" border=""0"" cellpadding=""0"" cellspacing=""0"" role=""presentation"" style=""background:#fffffe;background-color:#fffffe;width:100%;"">
<tbody><tr><td style=""border:none;direction:ltr;font-size:0;padding:0px 32px 32px 32px;text-align:center;"">";
var articleClosingTag = "</td></tr></tbody></table></div>";
var emailTemplate = File.ReadAllText(context.SharedFiles["NewsMonitor.zip/emailTemplate.html"]);
var insertCompany = File.ReadAllText(context.SharedFiles["NewsMonitor.zip/insertCompanyTag.txt"]);
var insertArticle = File.ReadAllText(context.SharedFiles["NewsMonitor.zip/insertArticleTag.txt"]);
var insertSeparator = File.ReadAllText(context.SharedFiles["NewsMonitor.zip/insertArticleSeparatorTag.txt"]);
var currentDate = DateTime.UtcNow.ToString("MM/dd/yy");
var csv_read_all = context.ScriptUtilities.LoadCsvFileIntoDataTable(csvPath);
var articleScanned = csv_read_all.Rows.Count;
DataView dv = new DataView(csv_read_all);
dv.RowFilter = "TRIM([Sentiment]) = 'negative'";
dv.Sort = "Company ASC";
DataTable filtered_data = dv.ToTable(true);
var negativeArticles = filtered_data.Rows.Count;
foreach (System.Data.DataRow row in filtered_data.Rows)
{
var companyName = row["Company"].ToString();
if (!negativeNewsArticles.ContainsKey(companyName))
negativeNewsArticles[companyName] = new List<Dictionary<string, string>>();
Dictionary<string, string> articleList = new Dictionary<string, string>
{
{"Title", row["Title"].ToString()},
{"Site Name", row["Site Name"].ToString()},
{"ImageURL", row["ImageURL"].ToString()},
{"TitleURL", row["Page Url"].ToString()}
};
negativeNewsArticles[companyName].Add(articleList);
}
foreach (var kvp in negativeNewsArticles)
{
List<string> articleMessageList = new List<string>();
var companyName = insertCompany.Replace("{COMPANY_NAME}", kvp.Key);
emailMessageList.Add(companyName);
foreach (var innerDict in kvp.Value)
{
var article = insertArticle.Replace("{ARTICLE_TITLE}", innerDict["Title"])
.Replace("{ARTICLE_SITE_NAME}", innerDict["Site Name"])
.Replace("{ARTICLE_IMAGE_URL}", innerDict["ImageURL"])
.Replace("{ARTICLE_TITLE_URL}", innerDict["TitleURL"]);
articleMessageList.Add(article);
}
var articleBody = string.Join(insertSeparator, articleMessageList.ToArray());
if (!string.IsNullOrEmpty(articleBody))
{
emailMessageList.Add(string.Format("{0}{1}{2}", articleOpeningTag, articleBody, articleClosingTag));
}
}
var articleData = string.Join("", emailMessageList.ToArray());
//context.Log("articleData value= "+articleData.ToString());
emailTemplate = emailTemplate.Replace("{CURRENT_DATE}", currentDate).Replace("{NUMBER_OF_NEGATIVE_ARTICLE}", negativeArticles.ToString()).Replace("{ARTICLE_SCANNED}", articleScanned.ToString()).Replace("{ARTICLE_DATA}", articleData);
//context.Log("emailTemplate value= "+emailTemplate.ToString());
return emailTemplate;
}
Timeout: This option allows you to customize the amount of time an agent waits for a script to get executed before proceeding. By default, this value is set to 10 minutes, ensuring compatibility with even slower systems and network connections. However, you can often reduce this timeout value to expedite the extraction process without compromising content accuracy.
To adjust the timeout value, simply select the desired duration from the dropdown menu within the script command. Available options include 2, 10, and 30 seconds; 1, 10, and 30 minutes; and 1 and 10 hours. Carefully consider your specific use case and the performance of your system when choosing the appropriate timeout value. By optimizing this setting, you can potentially enhance the efficiency of your script execution & agent-run tasks.

Scripting Area: This Area gives you the ability to write your script. This area changes as you change the selection of the “Language” and display relevant functions as per language to write scripting.
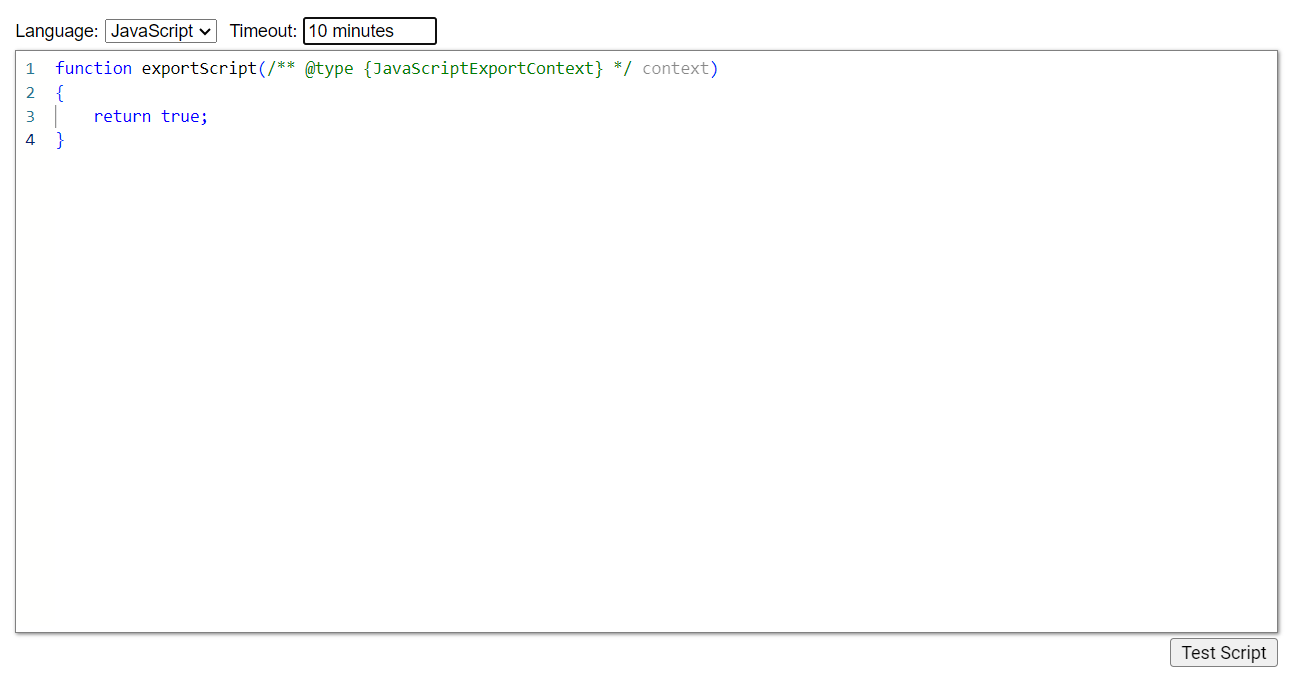
Files
Please refer to the “FIles” section for more insight.